|
| 1 | +# BIPEDAL ROBOT |
| 2 | + |
| 3 | +## CONTENTS |
| 4 | + |
| 5 | +- [Objective](#objective) |
| 6 | +- [Tasks](#tasks) |
| 7 | + - [Task 1 : Cruise Controller](#task-1-cruise-controller) |
| 8 | + - [Task 2 : Forward & Inverse Kinematics Solutions](#task-2-forward--inverse-kinematics) |
| 9 | + - [Task 3 : Trajectory Tracing](#task-3-trajectory-tracing) |
| 10 | +- [Project Implementation](#project-implementation) |
| 11 | +- [Design](#design) |
| 12 | +- [Electronic Components](#electronic-components) |
| 13 | +- [Final Implementation](#final-implementation) |
| 14 | +- [References](#references) |
| 15 | + |
| 16 | +## OBJECTIVE |
| 17 | +This project aims to develop a bipedal robot, a two-legged robotic system designed to replicate human gait dynamics. Here, the robot’s movement is controlled by precise limb positioning through inverse kinematics, while stability is maintained by managing the center of mass during walking. This repository provides an overview of the fundamental concepts, codes and model for simulating and executing stable bipedal locomotion, with an emphasis on kinematics and balance control. |
| 18 | + |
| 19 | +## TASKS |
| 20 | + |
| 21 | +### Task 1: Cruise Controller |
| 22 | +#### Aim |
| 23 | +To develop a Cruise Controller using Python and Matlab(simulink) for maintaining desired set point velocity of a car. |
| 24 | + |
| 25 | +**Simulink circuit design:** |
| 26 | + |
| 27 | + |
| 28 | + |
| 29 | +#### Result |
| 30 | + |
| 31 | +The PID controller was successfully tuned to achieve: |
| 32 | +- A rise time of approximately 10 seconds. |
| 33 | +- A maximum overshoot of less than 5%. |
| 34 | + |
| 35 | +**Python result:** |
| 36 | + |
| 37 | + |
| 38 | +**Simulink result:** |
| 39 | +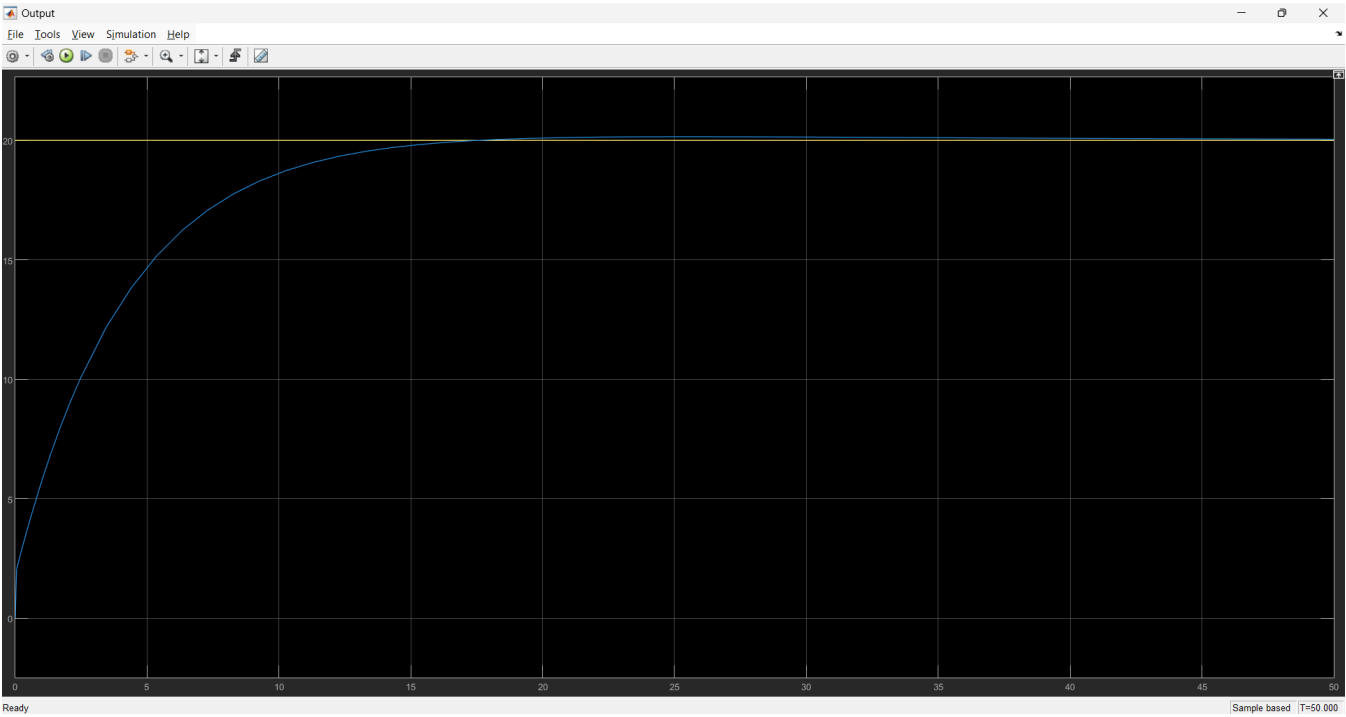 |
| 40 | + |
| 41 | +--- |
| 42 | + |
| 43 | +### Mechanics and Control of Robotic Manipulators |
| 44 | + |
| 45 | +In bipedal robots, kinematics plays a crucial role in defining and controlling movement. Kinematics is divided into forward kinematics and inverse kinematics, both of which are essential for achieving precise and coordinated motion in robotic manipulators. |
| 46 | + |
| 47 | +- **Forward Kinematics:** Forward kinematics involves calculating the robot's end-effector position based on given joint angles. In a bipedal robot, forward kinematics is used to determine the exact position of each leg segment when joint angles are set, enabling accurate placement of the feet during walking. |
| 48 | + |
| 49 | +- **Inverse Kinematics:** Inverse kinematics is the reverse process, where the desired position of the end-effector (such as the foot) is specified, and the necessary joint angles are calculated to achieve that position. This is particularly important in bipedal robots for defining precise leg trajectories that maintain stability and allow for smooth, balanced walking motions. |
| 50 | + |
| 51 | +### Task 2: Forward & Inverse Kinematics |
| 52 | +#### Aim |
| 53 | +To find FK/IK solutions of Manipulator arm in XY Plane in Python Using matplotlib |
| 54 | +#### Forward Kinematics Result: |
| 55 | +- Successfully defined the DH parameters and transformation matrices. |
| 56 | +- The forward kinematics function accurately calculates the end-effector position for the specified assembly. |
| 57 | + |
| 58 | + |
| 59 | + |
| 60 | + |
| 61 | + |
| 62 | +#### Inverse Kinematics Result: |
| 63 | +- Successfully defined the DH parameters and transformation matrices. |
| 64 | +- The inverse kinematics function accurately calculates the necessary joint angles for the specified end-effector position. |
| 65 | + |
| 66 | + |
| 67 | + |
| 68 | + |
| 69 | + |
| 70 | +### Task 3: Trajectory Tracing |
| 71 | +#### Aim |
| 72 | +To simulate 'a manipulator arm tracing a trajectory' in the XYZ plane using Python and Matplotlib. |
| 73 | +#### Result |
| 74 | +- Successfully simulated the arm manipulator in the XYZ plane using the chosen method. |
| 75 | +- The IK parameters were accurately determined, and the manipulator followed the intended trajectory in the simulation. |
| 76 | + |
| 77 | + |
| 78 | + |
| 79 | + |
| 80 | + |
| 81 | +--- |
| 82 | + |
| 83 | +## PROJECT IMPLEMENTATION |
| 84 | +- We decided to make 2dof manipulator as Bipedal Robot legs. |
| 85 | +- Formed the inverse kinematics equations and solved them on the Sinusoidal trajectory followed by retracment of Straight Line trajectory backwards. |
| 86 | +- Here is the python pseudocode for finding ik solutions: |
| 87 | +```python |
| 88 | +def inverse_kinematics(x, y): |
| 89 | + r = sqrt(x**2 + y**2) |
| 90 | + |
| 91 | + if r > (a1 + a2): |
| 92 | + raise ValueError("Target is not reachable") |
| 93 | + |
| 94 | + cos_theta2 = (r**2 - a1**2 - a2**2) / (2 * a1 * a2) |
| 95 | + cos_theta2 = np.clip(cos_theta2, -1.0, 1.0) |
| 96 | + sin_theta2 = sqrt(1 - cos_theta2**2) |
| 97 | + theta2 = atan2(sin_theta2, cos_theta2) |
| 98 | + |
| 99 | + theta1 = atan2(y, x) - atan2(a2 * sin(theta2), a1 + a2 * cos(theta2)) |
| 100 | + |
| 101 | +``` |
| 102 | + |
| 103 | +#### Result |
| 104 | +- Successfully simulated the 2DoF in XY Plane according to the required trajectory. |
| 105 | + |
| 106 | + |
| 107 | + |
| 108 | +--- |
| 109 | + |
| 110 | + |
| 111 | +## DESIGN |
| 112 | +- We used Autodesk Fusion 360 Software to create the CAD model for our 2DoF robot. |
| 113 | + |
| 114 | + |
| 115 | + |
| 116 | +--- |
| 117 | + |
| 118 | +## ELECTRONIC COMPONENTS |
| 119 | +We used 3 main components in our project. |
| 120 | + |
| 121 | +| **Component** | **Application** | |
| 122 | +|---------------|------------------------------------------| |
| 123 | +| Arduino UNO | Used Arduino UNO to connect all electronic equipments. | |
| 124 | +| Servo motors | Placed 6 servos with 3 servos on each leg: one in hip, one in knee and one in foot (to balance the COM while the robot is walking). | |
| 125 | +| PCA9685 | Used PCA9685 (with a 5V power supply) to connect and power multiple servo motors easily. | |
| 126 | +--- |
| 127 | + |
| 128 | +## FINAL IMPLEMENTATION |
| 129 | + |
| 130 | + |
| 131 | + |
| 132 | +## REFERENCES |
| 133 | +- https://www.youtube.com/playlist?list=PLyqSpQzTE6M-tWPjnJjFo9sHGWxgCnGrh |
| 134 | +- https://youtube.com/playlist?list=PLn8PRpmsu08pQBgjxYFXSsODEF3Jqmm-y&si=w728cftgoCuM-PjS |
| 135 | +- https://youtube.com/playlist?list=PLUMWjy5jgHK1NC52DXXrriwihVrYZKqjk&si=36dh-z4ip2kWO_XO |
0 commit comments