|
| 1 | +#!/usr/local/bin/python3 |
| 2 | + |
| 3 | +import os |
| 4 | +import glob |
| 5 | +import fileinput |
| 6 | +import linecache |
| 7 | +from functools import partial |
| 8 | + |
| 9 | +repo_dir = os.getcwd() |
| 10 | +source_dir = os.path.join(repo_dir, "source") |
| 11 | +all_md_path = os.path.join(repo_dir, "python-guide.pdf",) |
| 12 | + |
| 13 | +count = 0 |
| 14 | + |
| 15 | +with open(all_md_path, "w") as all_md: |
| 16 | + write = partial(print, file=all_md, end="") |
| 17 | + os.chdir(source_dir) |
| 18 | + |
| 19 | + for c_no in sorted(glob.glob("c*")): |
| 20 | + if c_no == "chapters" or c_no == "conf.py": |
| 21 | + continue |
| 22 | + |
| 23 | + # 读取并记下章节名 |
| 24 | + c_name = linecache.getline(os.path.join(source_dir, "chapters", f"{c_no.replace('c', 'p')}.rst"), 2) |
| 25 | + write(f"# {c_name}\n\n", file=all_md) |
| 26 | + |
| 27 | + # 读取每一节的内容 |
| 28 | + all_md_file = sorted(glob.glob(f"{source_dir}/{c_no}/*.md")) |
| 29 | + for line in fileinput.input(all_md_file): |
| 30 | + if "20200804124133" in line or "20200607174235" in line: |
| 31 | + continue |
| 32 | + |
| 33 | + if fileinput.isfirstline(): |
| 34 | + count += 1 |
| 35 | + if count%5 == 0: |
| 36 | + write("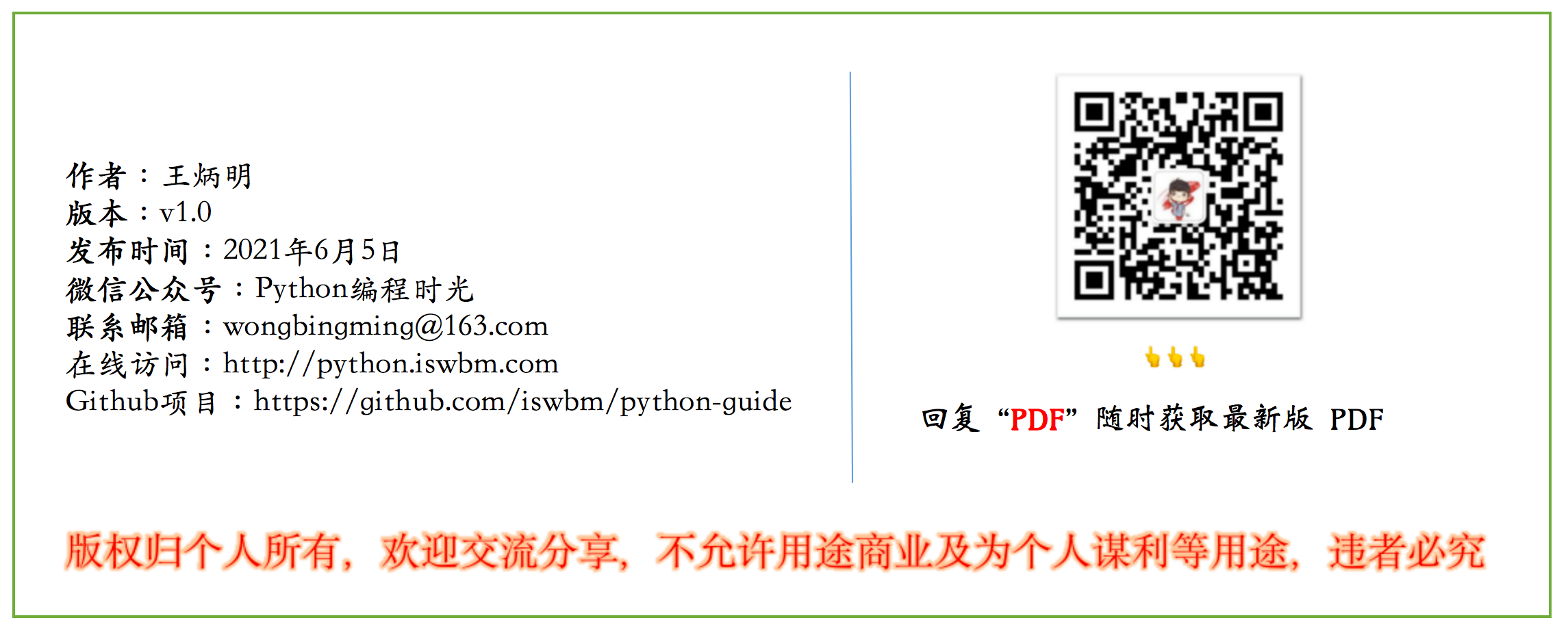", end="\n\n") |
| 37 | + |
| 38 | + if line.startswith("# "): |
| 39 | + line = line.replace("# ", "## ") |
| 40 | + elif line.startswith("## "): |
| 41 | + line = line.replace("## ", "### ") |
| 42 | + elif line.startswith("### "): |
| 43 | + line = line.replace("### ", "#### ") |
| 44 | + elif "gif" in line: |
| 45 | + line = line.replace("![]", "![该图为GIF,请前往 python.iswbm.com 浏览]") |
| 46 | + |
| 47 | + write(line) |
| 48 | + |
0 commit comments