Commit 53b4b40
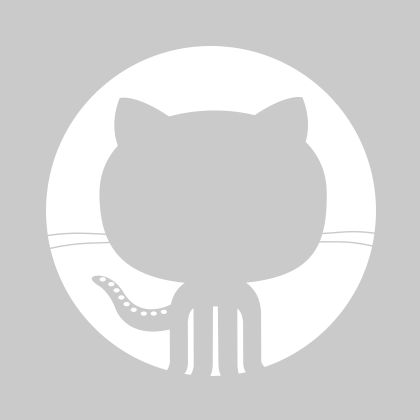
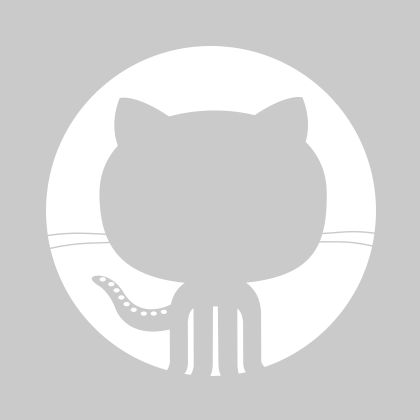
Yair Even Or
Yair Even Or
1 parent 93b7ff8 commit 53b4b40
File tree
9 files changed
+503
-73
lines changed- dist
- src
- utils
9 files changed
+503
-73
lines changed+18
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
153 | 153 |
| |
154 | 154 |
| |
155 | 155 |
| |
| 156 | + | |
| 157 | + | |
| 158 | + | |
| 159 | + | |
| 160 | + | |
| 161 | + | |
| 162 | + | |
| 163 | + | |
| 164 | + | |
| 165 | + | |
| 166 | + | |
| 167 | + | |
| 168 | + | |
| 169 | + | |
| 170 | + | |
| 171 | + | |
| 172 | + | |
| 173 | + |
+425-2
Large diffs are not rendered by default.
+22-10
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
93 | 93 |
| |
94 | 94 |
| |
95 | 95 |
| |
| 96 | + | |
| 97 | + | |
96 | 98 |
| |
97 | 99 |
| |
98 | 100 |
| |
99 | 101 |
| |
100 |
| - | |
| 102 | + | |
| 103 | + | |
101 | 104 |
| |
102 | 105 |
| |
103 |
| - | |
| 106 | + | |
104 | 107 |
| |
105 | 108 |
| |
106 | 109 |
| |
107 |
| - | |
| 110 | + | |
108 | 111 |
| |
109 | 112 |
| |
110 | 113 |
| |
111 |
| - | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
112 | 117 |
| |
113 |
| - | |
| 118 | + | |
114 | 119 |
| |
115 | 120 |
| |
116 | 121 |
| |
117 | 122 |
| |
118 | 123 |
| |
119 | 124 |
| |
120 |
| - | |
121 |
| - | |
| 125 | + | |
| 126 | + | |
122 | 127 |
| |
123 | 128 |
| |
124 | 129 |
| |
| |||
129 | 134 |
| |
130 | 135 |
| |
131 | 136 |
| |
132 |
| - | |
133 |
| - | |
| 137 | + | |
| 138 | + | |
134 | 139 |
| |
135 | 140 |
| |
136 | 141 |
| |
137 | 142 |
| |
138 | 143 |
| |
139 | 144 |
| |
140 | 145 |
| |
141 |
| - | |
| 146 | + | |
142 | 147 |
| |
| 148 | + | |
| 149 | + | |
143 | 150 |
| |
| 151 | + | |
| 152 | + | |
| 153 | + | |
| 154 | + | |
| 155 | + | |
144 | 156 |
| |
145 | 157 |
| |
146 | 158 |
| |
|
+1-38
Some generated files are not rendered by default. Learn more about customizing how changed files appear on GitHub.
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
11 | 11 |
| |
12 | 12 |
| |
13 | 13 |
| |
| 14 | + | |
14 | 15 |
| |
15 | 16 |
| |
16 | 17 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
15 | 15 |
| |
16 | 16 |
| |
17 | 17 |
| |
| 18 | + | |
| 19 | + | |
18 | 20 |
| |
19 | 21 |
| |
20 | 22 |
| |
|
+15-19
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
| 7 | + | |
8 | 8 |
| |
9 | 9 |
| |
10 | 10 |
| |
| |||
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
22 |
| - | |
| 21 | + | |
23 | 22 |
| |
24 | 23 |
| |
25 | 24 |
| |
| |||
39 | 38 |
| |
40 | 39 |
| |
41 | 40 |
| |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 | 41 |
| |
56 | 42 |
| |
57 | 43 |
| |
58 | 44 |
| |
59 | 45 |
| |
60 | 46 |
| |
61 | 47 |
| |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
62 | 54 |
| |
63 | 55 |
| |
64 | 56 |
| |
| |||
128 | 120 |
| |
129 | 121 |
| |
130 | 122 |
| |
131 |
| - | |
132 |
| - | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
133 | 127 |
| |
134 |
| - | |
| 128 | + | |
135 | 129 |
| |
136 | 130 |
| |
137 | 131 |
| |
| |||
175 | 169 |
| |
176 | 170 |
| |
177 | 171 |
| |
| 172 | + | |
| 173 | + | |
178 | 174 |
|
+1-4
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
| 4 | + | |
8 | 5 |
| |
9 | 6 |
| |
10 | 7 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
70 | 70 |
| |
71 | 71 |
| |
72 | 72 |
| |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + |
0 commit comments